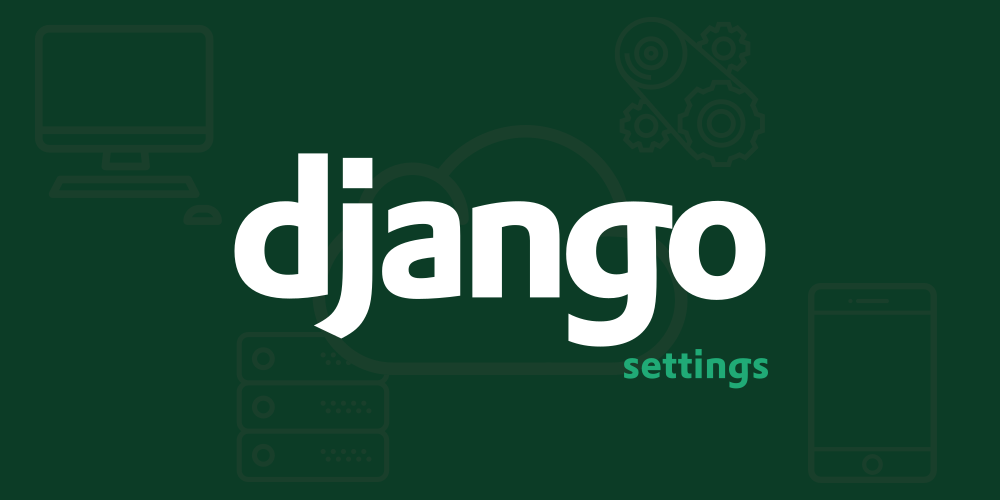
Using environment variables with Django
It is important to keep sensitive bits of code like API keys and database credentials away from others. The best way to do this is to not put them on GitHub! Even if you are developing a personal project with no real users, securing your environment variables will build good habits and prevent annoying emails from GitGuardian. Here’s how to do it in Django.
0. Install Django Environ
In your terminal, inside the project directory, type:
pip install django-environ
1. Initialize environ in you settings.py
import environ
# Initializing environment variables
env = environ.Env()
environ.Env.read_env()
2. Create your .env file
In the same directory as settings.py, create a file called ‘.env’
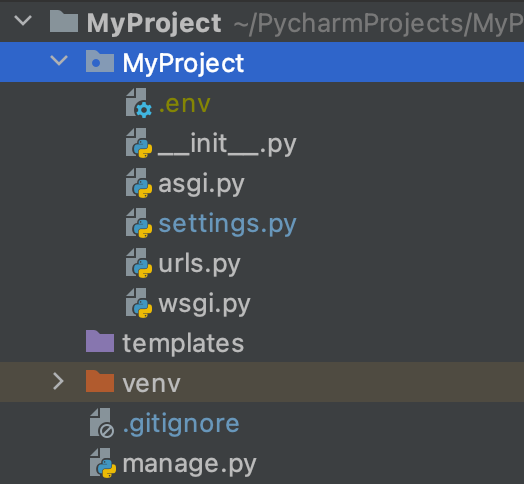
Make sure you don’t use quotations around strings.
DEBUG=True
SECRET_KEY=django-insecure-pn_5^6d^c&)9x@_1tsw!4a+*#!_rgtaej#&ss!4il0a-!97p&h
ALLOWED_HOSTS=elkhayyat.me,localhost:8000
DB_HOST=localhost
DB_PORT=3306
DB_NAME=my_project
DB_USER=elkhayyat
DB_PASSWORD=ELKHAYYATdotME
3. IMPORTANT: Add your .env file to .gitignore
4. Replace all references to your environment variables in settings.py
DEBUG = True if str(env('DEBUG')).upper() == "TRUE" else False
SECRET_KEY = env('SECRET_KEY')
# Splitting ALLOWED_HOSTS by comma
# NOTE:: do not place spaces after comma in .env
ALLOWED_HOSTS = env('ALLOWED_HOSTS').split(',')
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': env('DB_NAME'),
'USER': env('DB_USER'),
'PASSWORD': env('DB_PASSWORD'),
'HOST': env('DB_HOST'), # Or an IP Address that your DB is hosted on
'PORT': env('DB_PORT'),
}
}
TEST
Let’s test it by migrating and run server.
python manage.py migrate
python manage.py runserver
Thanks for reading.
Leave a Reply