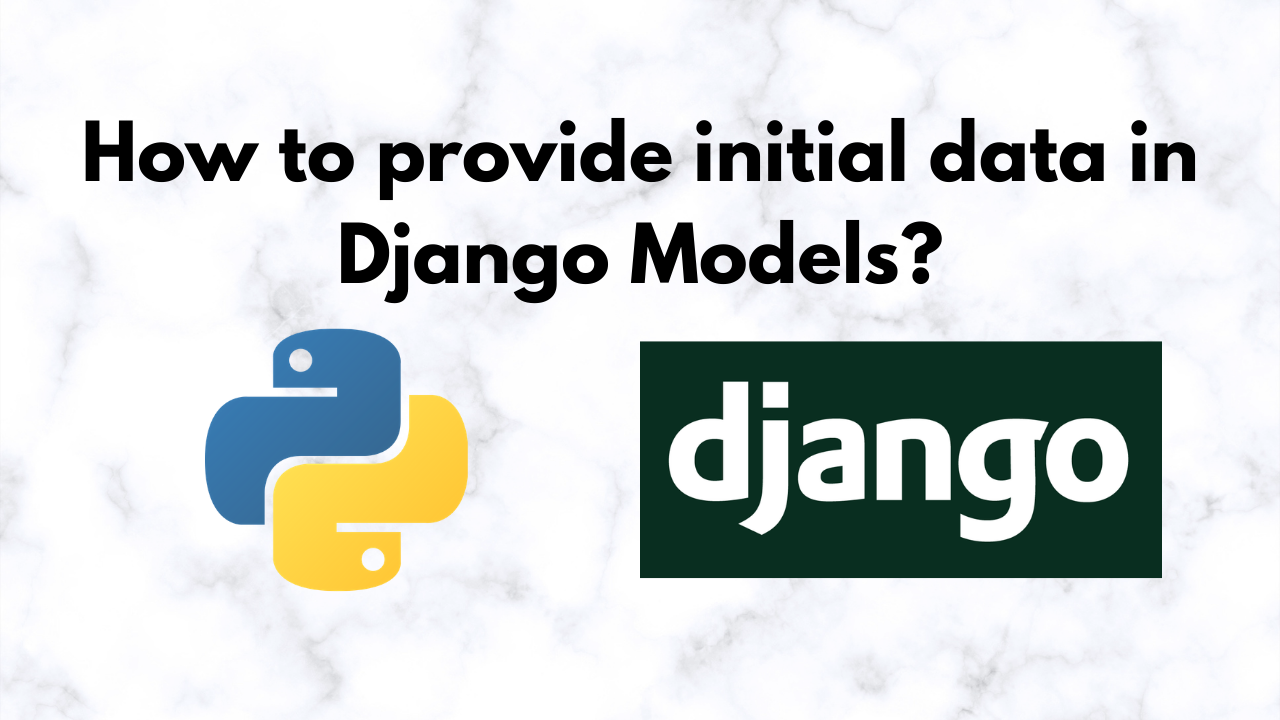
How to add initial data to your Django project?
1- Run this command and change myapp to your app name:
python manage.py makemigrations --empty myapp
This command will create an empty migration file inside your app migrations directory, it will look like the following.
# Generated by Django 4.2.2 on 2023-06-15 10:15
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
("myapp", "0001_initial"),
]
operations = []
2- Write your initial data as a list of dicts.
INITIAL_DATA = [
{
"parent_type": "car",
"label": "Sedan",
"need_license_plate": True,
},
{
"parent_type": "car",
"label": "Cargo van",
"need_license_plate": True,
},
{
"parent_type": "bike",
"label": "Moped Bike",
"need_license_plate": True,
},
{
"parent_type": "bike",
"label": "E-Bike",
"need_license_plate": True,
},
]
3- Create a function called load_initial_data to add the data into the database
def load_initial_data(apps, schema_editor):
vehicle_types_model = apps.get_model('myapp', 'VehicleType')
for data in INITIAL_DATA:
vehicle_types_model.objects.create(**data)
Let me explain what this function does
vehicle_types_model = apps.get_model('myapp', 'VehicleType')
at this line, we are identifying the app name and the model name so you will replace myapp with your app_name and VehicleType with your model_name
for data in INITIAL_DATA:
vehicle_types_model.objects.create(**data)
This for loop is to loop over the INITIAL_DATA and add it into the database
3- Add this item to the operations list
operations = [
migrations.RunPython(load_initial_data)
]
This command runs the load_initial_data function when you run your migration file
The final file will look like the following
# Generated by Django 4.2.2 on 2023-06-15 10:15
from django.db import migrations
INITIAL_DATA = [
{
"parent_type": "car",
"label": "Sedan",
"need_license_plate": True,
},
{
"parent_type": "car",
"label": "SUV",
"need_license_plate": True,
},
{
"parent_type": "car",
"label": "Minivan",
"need_license_plate": True,
},
{
"parent_type": "car",
"label": "Cargo van",
"need_license_plate": True,
},
{
"parent_type": "car",
"label": "Pickup truck",
"need_license_plate": True,
},
{
"parent_type": "car",
"label": "Box truck",
"need_license_plate": True,
},
{
"parent_type": "car",
"label": "Other",
"need_license_plate": True,
},
{
"parent_type": "bike",
"label": "Moped Bike",
"need_license_plate": True,
},
{
"parent_type": "bike",
"label": "E-Bike",
"need_license_plate": True,
},
{
"parent_type": "bike",
"label": "Manual Bike",
"need_license_plate": False,
},
]
def load_initial_data(apps, schema_editor):
vehicle_types_model = apps.get_model('vehicles', 'VehicleType')
for data in INITIAL_DATA:
vehicle_types_model.objects.create(**data)
class Migration(migrations.Migration):
dependencies = [
("myapp", "0001_initial"),
]
operations = [
migrations.RunPython(load_initial_data)
]
Thanks for reading 😉
AHMED ELKHAYYAT
Leave a Reply